Why DBOS?
DBOS is an open-source library for building reliable and fault-tolerant applications.
Let's look at a common situation where reliability is critical. Imagine you're running an e-commerce platform where an order goes through multiple steps:
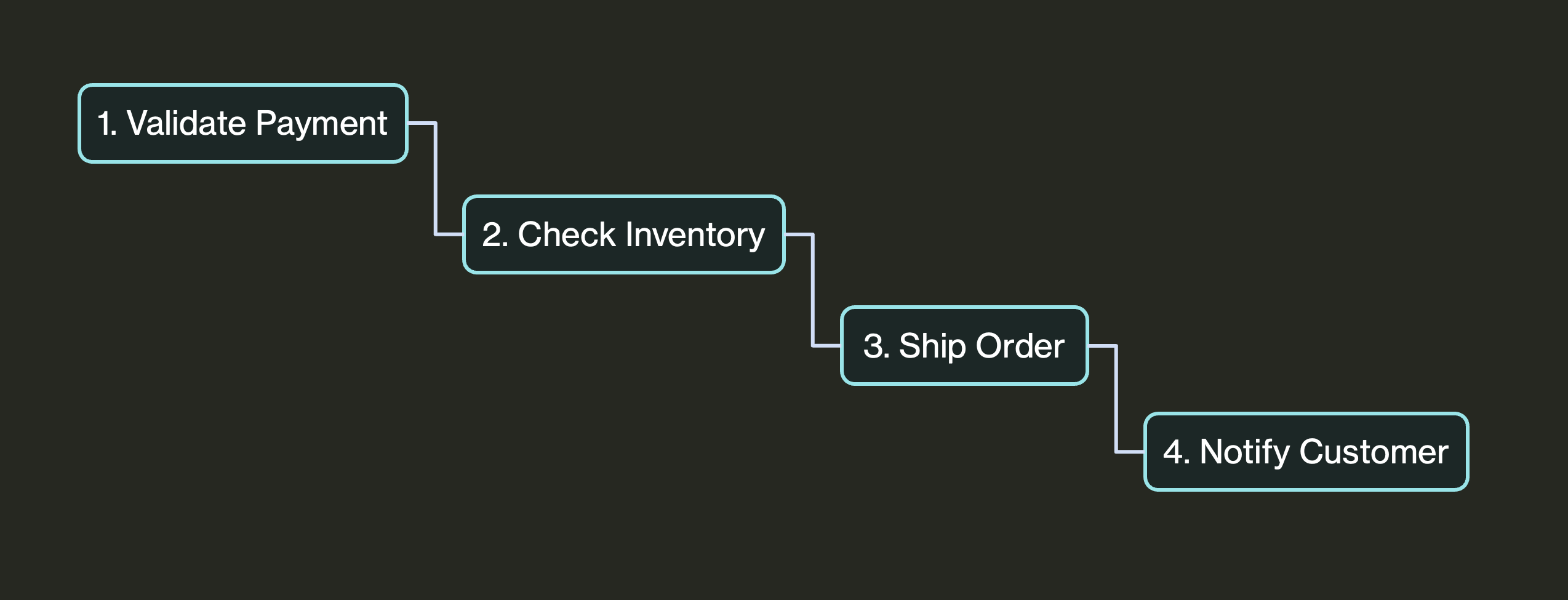
This program looks simple, but making it reliable is deceptively difficult. Here are two out of many potential problems:
- Your program crashes after step 1, "Validate Payment". The customer has been charged, but their order is never shipped.
- You get to step 2, "Check Inventory", and you're out of stock. You need to wait 24 hours for the new inventory before you can ship your order. You need that step to sleep for a day.
These situations can cause hours (or even days!) of debugging and may require deep distributed systems expertise to solve.
DBOS makes them easier to solve because you can add decorators like DBOS.workflow()
and DBOS.step()
to your program:
@DBOS.step()
def validate_payment():
...
@DBOS.workflow()
def checkout_workflow()
validate_payment()
check_inventory()
ship_order()
notify_customer()
These decorators durably execute your program, persisting its state to a Postgres database:
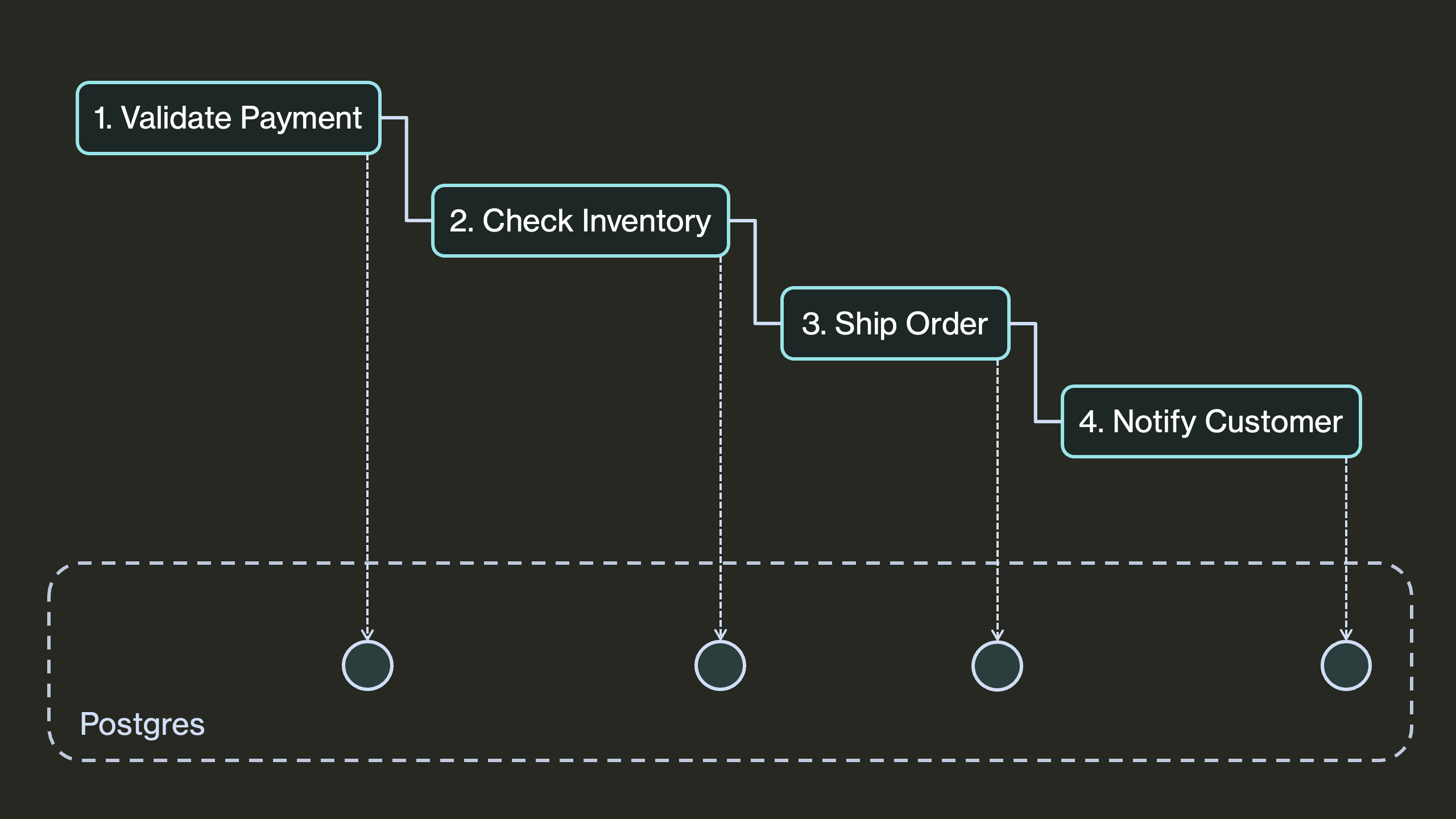
You can think of this stored state as a checkpoint for your program. If your program is ever interrupted or crashes, DBOS uses this saved state to recover it from the last completed step. For example, if your checkout workflow crashes right after validating payment, instead of the order being lost forever, DBOS recovers from a checkpoint and goes on to ship the order. Thus, DBOS makes your application resilient to any failure.
DBOS Is Lightweight
All you need to use DBOS is install the open-source DBOS Transact library (Python, TypeScript).
You can run these commands to quickly try out a DBOS demo yourself:
- Python
- TypeScript
pip install dbos
dbos init
dbos start
npx @dbos-inc/create -t dbos-node-starter
cd dbos-node-starter
npm run build
npm run start
To add DBOS to your application, simply annotate workflows and steps in your program like this:
- Python
- TypeScript
@DBOS.step()
def step_one():
...
@DBOS.step()
def step_two():
...
@DBOS.workflow()
def workflow()
step_one()
step_two()
class Example {
@DBOS.step()
static async step_one() {
...
}
@DBOS.step()
static async step_two() {
...
}
@DBOS.workflow()
static async workflow() {
await Example.step_one()
await Example.step_two()
}
}
If your program is ever interrupted or crashed, all your workflows automatically resume from the last completed step.
Use Cases
DBOS helps you write complex programs in remarkably few lines of code. For example:
- Reliable Workflows
- Background Tasks
- Cron Jobs
- Data Pipelines
- Kafka
- AI Agents
Write business logic in normal code, with branches, loops, subtasks, and retries. DBOS makes it resilient to any failure.
@DBOS.step()
def validate_payment():
...
@DBOS.workflow()
def checkout_workflow()
validate_payment()
check_inventory()
ship_order()
notify_customer()
Launch any task to run in the background and guarantee it eventually completes. Wait for days or weeks, or for a notification, before continuing.
@DBOS.workflow()
def schedule_reminder(to_email, days_to_wait):
DBOS.recv(days_to_seconds(days_to_wait))
send_reminder_email(to_email, days_to_wait)
@app.post("/email")
def email_endpoint(request):
DBOS.start_workflow(schedule_reminder, request.email, request.days)
Schedule functions to run at specific times. Host them serverlessly on DBOS Cloud.
@DBOS.scheduled("0 * * * *") # Run once an hour
@DBOS.workflow()
def run_hourly(scheduled_time, actual_time):
results = search_hackernews("serverless")
for comment, url in results:
post_to_slack(comment, url)
Build data pipelines that are reliable and observable by default. DBOS durable queues guarantee all your tasks complete.
queue = Queue("indexing_queue")
@DBOS.workflow()
def indexing_workflow(urls):
handles = []
for url in urls:
handles.append(queue.enqueue(index_step, url))
return [h.get_result() for h in handles]
Consume Kafka messages exactly-once, no need to worry about timeouts or offsets.
@DBOS.kafka_consumer(config,["alerts-topic"])
@DBOS.workflow()
def process_kafka_alerts(msg):
alerts = msg.value.decode()
for alert in alerts:
respond_to_alert(alert)
Enhance your AI agents with reliable asynchronous tasks and human in the loop. Integrate with popular frameworks like LangChain and LlamaIndex.
@DBOS.workflow()
def agentic_refund(purchase):
email_human_for_approval(purchase)
status = DBOS.recv(timeout_seconds=APPROVAL_TIMEOUT)
if status == "approve":
approve_refund(purchase)
else:
reject_refund(purchase)
Thanks to Paul Copplestone from Supabase, whose blog post on DBOS inspired this page.