Cloud Cron Quickstart
Let's say you want to run some code on a schedule. For example, you want to:
- Record a stock's price once a minute.
- Migrate some data from one database to another once an hour.
- Send emails to inactive users once a week.
This kind of code isn't easy to manage because the server running it has to always be "on"—you can't just run it on your laptop.
In this tutorial, we'll show you how to use DBOS to run code on a schedule in the cloud so you don't have to worry about maintaining it. You'll learn how to write a scheduled (cron) function in just 11 lines of Python code and deploy it to the cloud with a single click.
Tutorial
1. Select the Cloud Cron Starter
Visit https://console.dbos.dev/launch and select the DBOS Cron Starter. When prompted, create a database for your app with default settings.
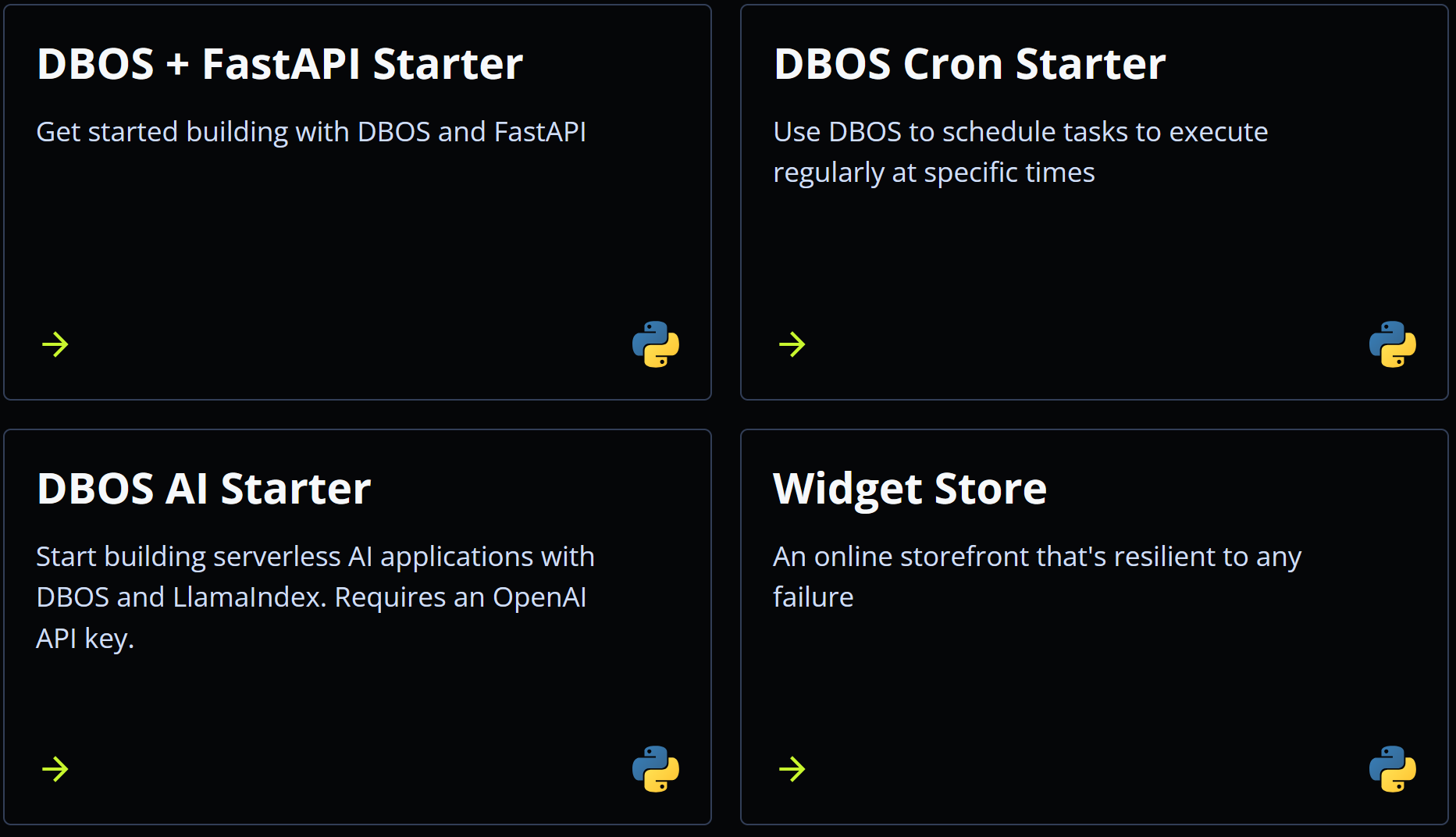
2. Connect to GitHub and Deploy to DBOS Cloud
To ensure you can easily update your project after deploying it, DBOS will create a GitHub repository for you. You can deploy directly from that GitHub repository to DBOS Cloud.
First, sign in to your GitHub account. Then, set your repository name and whether it should be public or private.
Next, click "Create GitHub Repo and Deploy" and DBOS will clone a copy of the source code into your GitHub account, then deploy your project to DBOS Cloud. In less than a minute, your app should deploy successfully.
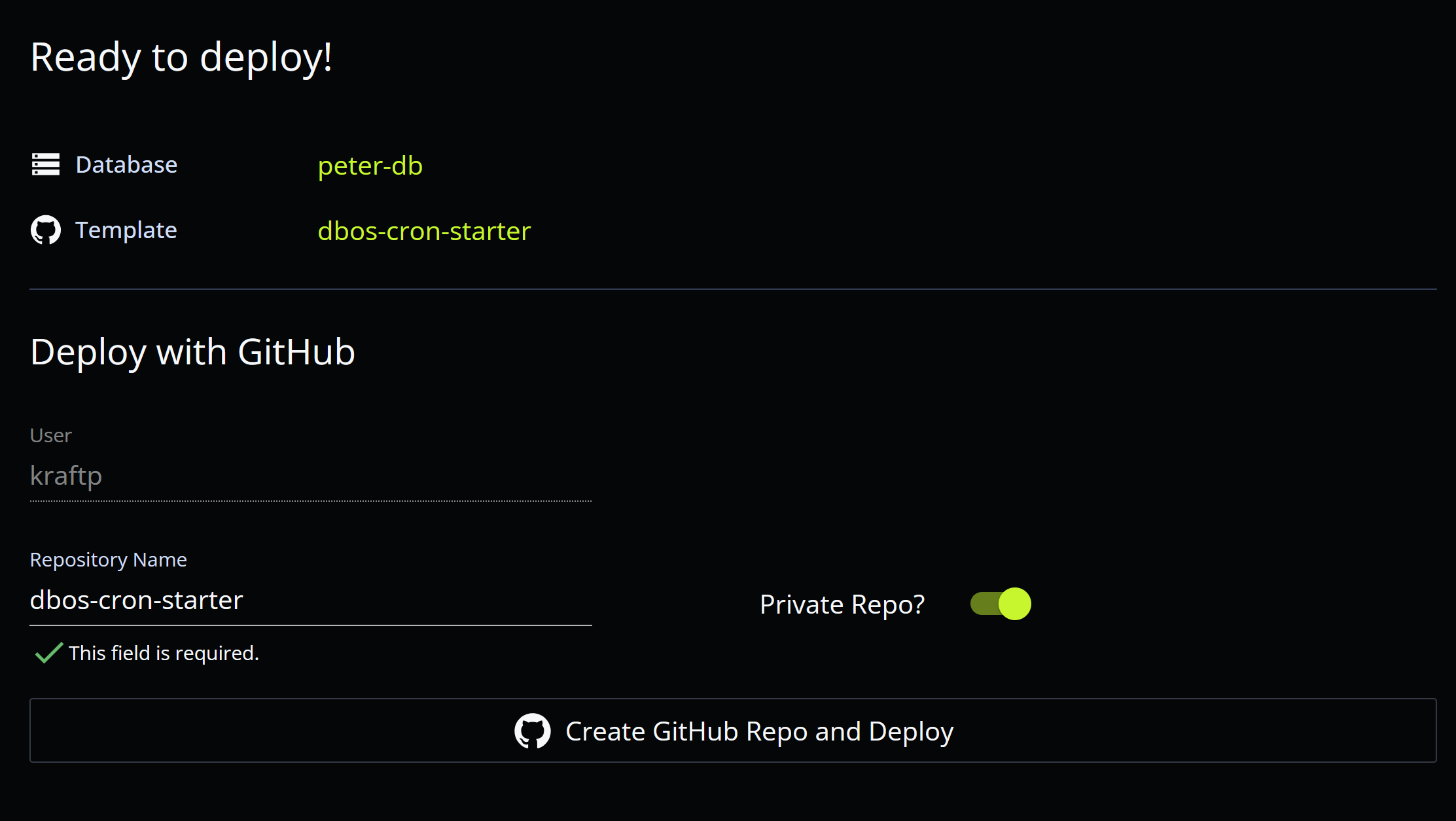
3. View Your Application
At this point, your app is running code on a schedule in the cloud!
To see its code, visit your new GitHub repository and open app/main.py
.
The app schedules a function incrementing a counter to run once a minute (the cron syntax * * * * *
means "once a minute").
You can visit your app's URL to see the current value of the counter.
from dbos import DBOS
from fastapi import FastAPI
app = FastAPI()
DBOS(fastapi=app)
counter = 0
@DBOS.scheduled("* * * * *")
@DBOS.step()
def scheduled_function(scheduled_time, actual_time):
global counter
counter += 1
@app.get("/")
def endpoint():
return f"The scheduled function has run {counter} times!"
4. Start Building
To start building, edit your application on GitHub (source code is in app/main.py
), commit your changes, then press "Deploy From GitHub" on your applications page to see your changes reflected in the live application.
Not sure where to start? Try adding this line to the scheduled function so it logs each time it runs:
DBOS.logger.info(f"I just ran at {scheduled_time}")
You can view your application's logs from your applications page.
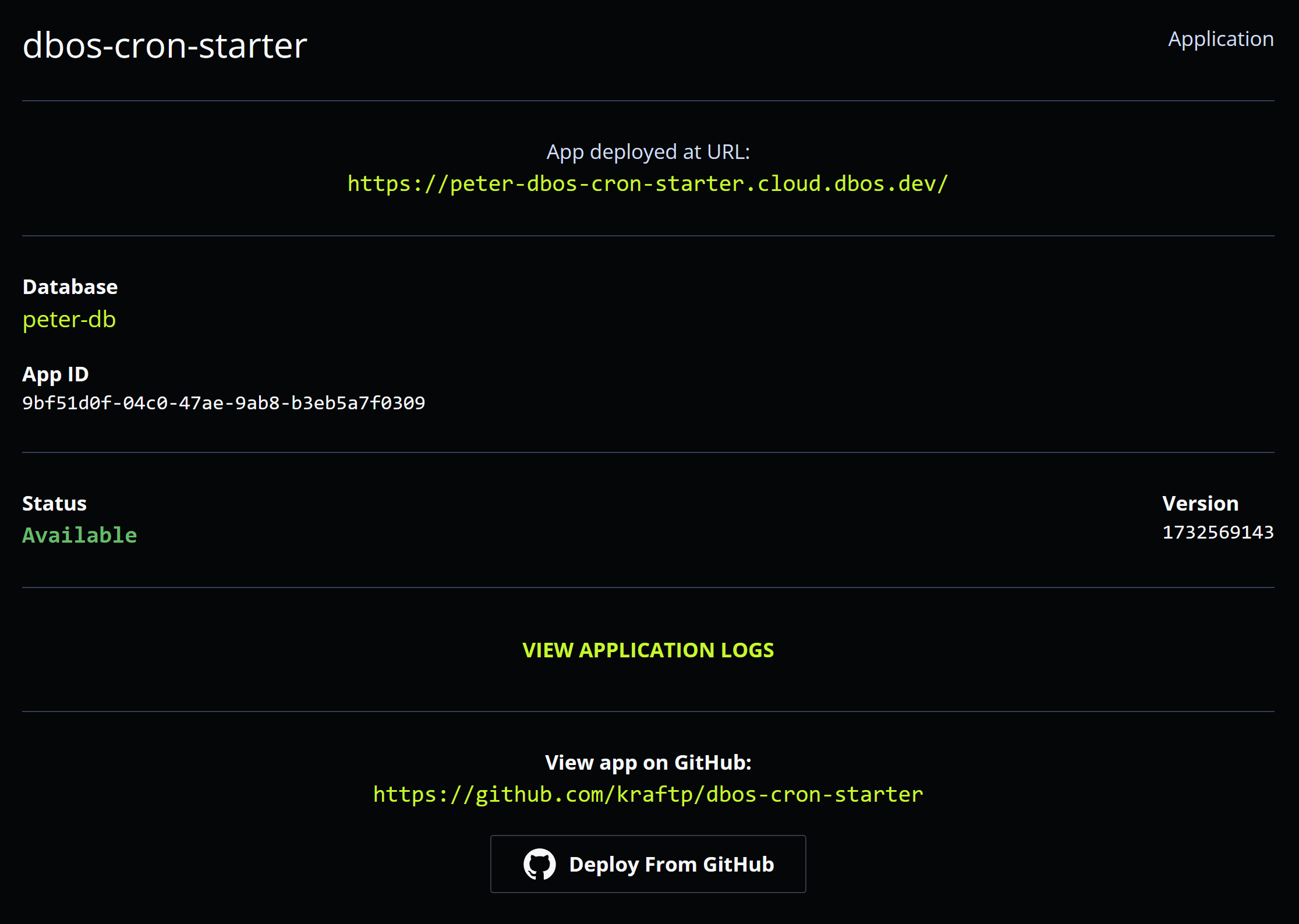
Next Steps
You can adapt this 6-line starter to implement your own scheduled job.
Replace scheduled_function
with your own function to run it on a schedule!
Some useful implementation notes:
- Schedules are specified in crontab syntax.
For example,
* * * * *
means "run once a minute." To learn more about crontab syntax, see this guide. - The two arguments passed into
scheduled_function
are the time the run was scheduled (as adatetime
) and the time the run was actually started (as adatetime
). - For more information, see the scheduling documentation.
Here are two larger examples built with DBOS scheduling:
- Hacker News Slackbot: Periodically search Hacker News for people commenting about serverless computing and post the comments to Slack.
- Earthquake Tracker: Use a scheduled job to scrape earthquake data from the USGS, then build a real-time earthquake dashboard over it.
Running It Locally
You can also run your application locally for development and testing.
1. Git Clone Your Application
Clone your application from git and enter its directory.
git clone <your-git-url>
cd dbos-cron-starter
2. Set up a virtual environment
Create a virtual environment and install dependencies.
- macOS or Linux
- Windows (PowerShell)
- Windows (cmd)
python3 -m venv .venv
source .venv/bin/activate
pip install -r requirements.txt
python3 -m venv .venv
.venv\Scripts\activate.ps1
pip install -r requirements.txt
python3 -m venv .venv
.venv\Scripts\activate.bat
pip install -r requirements.txt
3. Install the DBOS Cloud CLI
The Cloud CLI requires Node.js 20 or later.
Instructions to install Node.js
- macOS or Linux
- Windows
Run the following commands in your terminal:
curl -o- https://raw.githubusercontent.com/nvm-sh/nvm/v0.40.1/install.sh | bash
export NVM_DIR="$HOME/.nvm"
[ -s "$NVM_DIR/nvm.sh" ] && \. "$NVM_DIR/nvm.sh" # This loads nvm
nvm install 22
nvm use 22
Download Node.js 20 or later from the official Node.js download page and install it.
After installing Node.js, create the following folder: C:\Users\%user%\AppData\Roaming\npm
(%user%
is the Windows user on which you are logged in).
Run this command to install it.
npm i -g @dbos-inc/dbos-cloud@latest
4. Connect Your Application to Postgres
Under the hood, DBOS uses Postgres for scheduling, so you need to connect your app to a Postgres database—you can use a DBOS Cloud database, a Docker container, or a local Postgres installation:
Instructions to set up Postgres
- Use Cloud Postgres
- Launch Postgres with Docker
- Install Postgres
You can connect your local application to a Postgres database hosted in DBOS Cloud.
First, set a password for your DBOS Cloud database:
dbos-cloud db reset-password
Then connect your local app to your cloud database. When prompted, enter the password you just set.
dbos-cloud db local
- macOS
- Linux
- Windows (PowerShell)
- Windows (cmd)
You can install Docker on macOS through Docker Desktop.
Then, run this script to launch Postgres in a Docker container:
export PGPASSWORD=dbos
# Docker may require sudo -E
python3 start_postgres_docker.py
Follow the Docker Engine installation page to install Docker on several popular Linux distributions.
Then, run this script to launch Postgres in a Docker container:
export PGPASSWORD=dbos
# Docker may require sudo -E
python3 start_postgres_docker.py
You can install Docker on Windows through Docker Desktop.
Then, run this script to launch Postgres in a Docker container:
$env:PGPASSWORD = "dbos"
python3 start_postgres_docker.py
You can install Docker on Windows through Docker Desktop.
Then, run this script to launch Postgres in a Docker container:
set PGPASSWORD=dbos
python3 start_postgres_docker.py
If successful, the script should print Database started successfully!
- macOS
- Linux
- Windows (PowerShell)
- Windows (cmd)
Follow this guide to install Postgres on macOS.
Then, set the PGPASSWORD
environment variable to your Postgres password:
export PGPASSWORD=<your-postgres-password>
Follow these guides to install Postgres on popular Linux distributions.
Then, set the PGPASSWORD
environment variable to your Postgres password:
export PGPASSWORD=<your-postgres-password>
Follow this guide to install Postgres on Windows.
Then, set the PGPASSWORD
environment variable to your Postgres password:
$env:PGPASSWORD = "<your-postgres-password>"
Follow this guide to install Postgres on Windows.
Then, set the PGPASSWORD
environment variable to your Postgres password:
set PGPASSWORD=<your-postgres-password>
Alternatively, if you already have a Postgres database, update dbos-config.yaml
with its connection information.
5. Start Your Appliation
Start your application with dbos start
, then visit http://localhost:8000
to see it!
dbos start